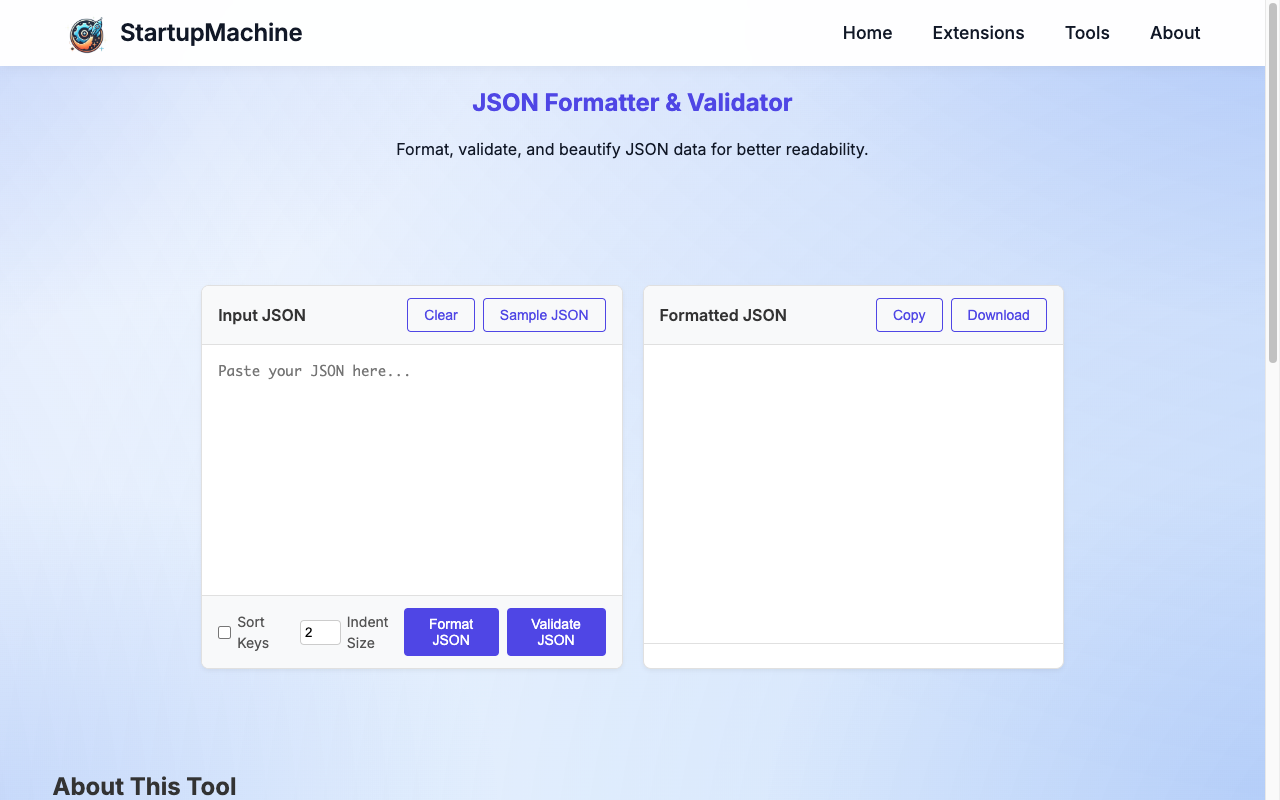
StartupMachine's JSON Formatter & Validator tool interface
JSON (JavaScript Object Notation) has become the universal language for data exchange on the web. Its simplicity and versatility make it the format of choice for APIs, configuration files, and data storage. However, working with JSON can sometimes be challenging, especially when dealing with complex structures or debugging syntax errors.
This is where a JSON formatter and validator becomes an essential tool in a developer's arsenal. Our JSON Formatter & Validator tool helps you properly structure, validate, and debug your JSON data, making your development process smoother and more efficient.
In this comprehensive guide, we'll explore how to use the JSON Formatter & Validator tool to format messy JSON, identify and fix syntax errors, and ensure your JSON data is valid and well-structured.
Key Takeaways
- Understand the common pitfalls and syntax requirements of JSON
- Learn how to properly format and beautify JSON data for readability
- Master JSON validation techniques to identify and fix syntax errors
- Discover advanced features like minification and tree view visualization
- Explore best practices for working with JSON in web development
Understanding JSON Basics
Before diving into the tool, let's review some JSON fundamentals:
JSON Structure and Syntax
JSON is built on two primary structures:
- Objects: Collections of name/value pairs enclosed in curly braces {}
- Arrays: Ordered lists of values enclosed in square brackets []
JSON values can be:
- A string (in double quotes)
- A number
- An object
- An array
- A boolean (true or false)
- null
Example of Valid JSON
{
"firstName": "John",
"lastName": "Doe",
"age": 30,
"isEmployee": true,
"contact": {
"email": "[email protected]",
"phone": "+1-555-555-5555"
},
"skills": ["JavaScript", "HTML", "CSS"],
"address": null
}
Did You Know?
Despite its name and origins in JavaScript, JSON is language-independent and can be used with virtually any programming language that can parse text and create data structures.
Common JSON Issues
Developers frequently encounter these JSON-related problems:
Syntax Errors
- Missing or mismatched brackets and braces
- Trailing commas (not allowed in JSON)
- Unquoted property names
- Single quotes instead of double quotes
- Missing commas between elements
Readability Problems
- Minified JSON that's difficult to read and debug
- Inconsistent indentation
- Extremely nested structures without proper formatting
Data Structure Issues
- Inconsistent data types
- Duplicate keys in objects
- Circular references (not supported in JSON)
Our JSON Formatter & Validator tool addresses these common issues by providing a simple way to check and format your JSON data.
Using the JSON Formatter & Validator
Accessing the Tool
Let's start by accessing our JSON Formatter & Validator tool. The interface is clean and straightforward, featuring a text area where you can paste your JSON data.
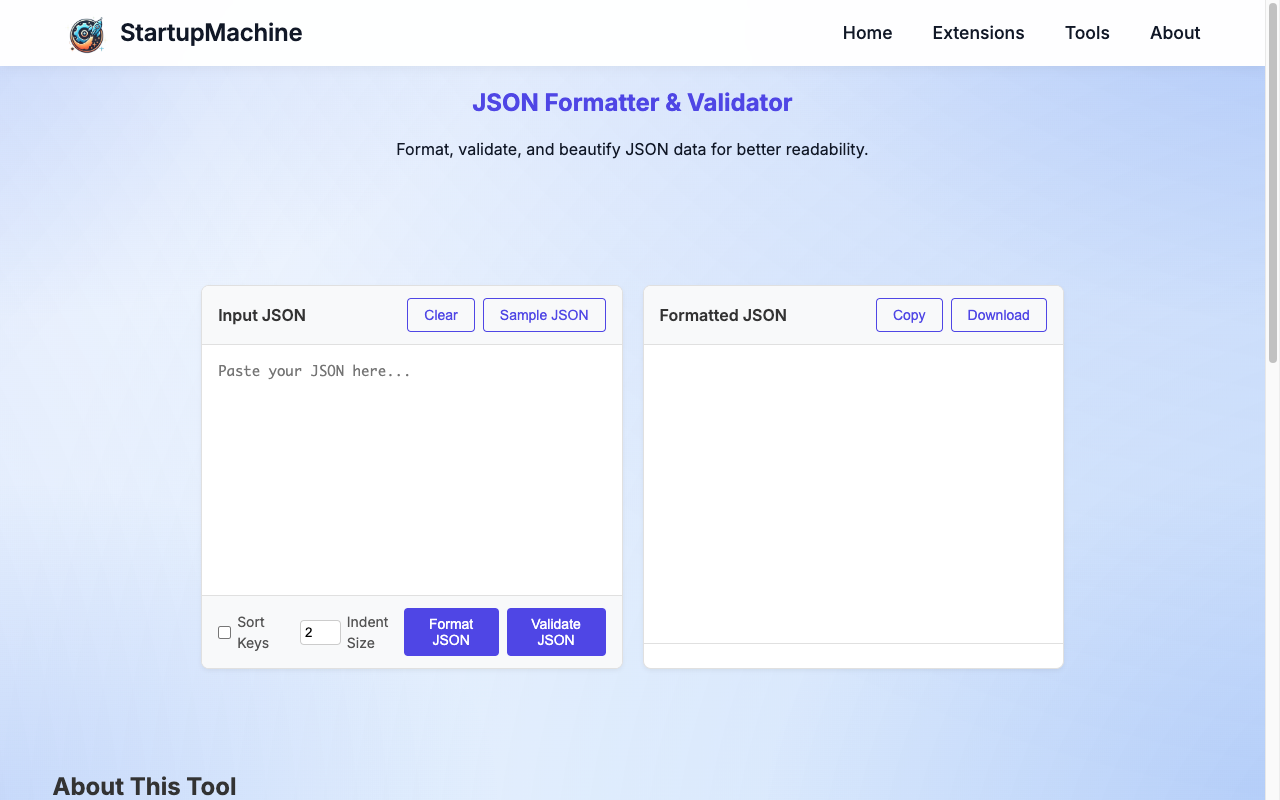
The JSON Formatter & Validator tool's main interface
Formatting JSON
To format your JSON data:
- Paste your JSON into the text area
- Click the "Format JSON" button
- The tool will automatically indent your JSON, making it more readable
- You can adjust the indentation level using the settings (2 spaces, 4 spaces, or tab)
Before Formatting (Minified JSON)
{"users":[{"id":1,"name":"John Smith","email":"[email protected]","active":true,"roles":["admin","editor"]},{"id":2,"name":"Jane Doe","email":"[email protected]","active":false,"roles":["viewer"]}],"total":2,"page":1}
After Formatting
{
"users": [
{
"id": 1,
"name": "John Smith",
"email": "[email protected]",
"active": true,
"roles": [
"admin",
"editor"
]
},
{
"id": 2,
"name": "Jane Doe",
"email": "[email protected]",
"active": false,
"roles": [
"viewer"
]
}
],
"total": 2,
"page": 1
}
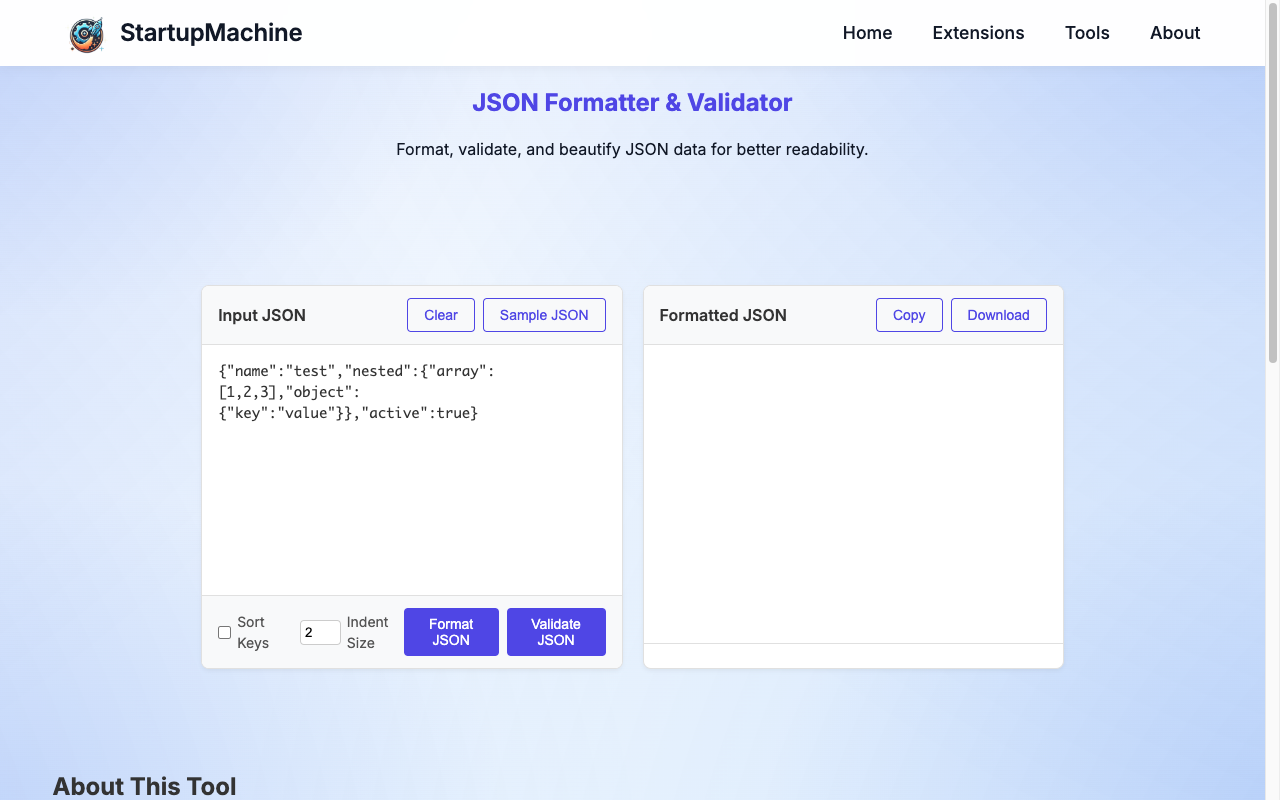
Properly formatted JSON with clear indentation
Pro Tip
For large JSON files, proper formatting not only improves readability but also helps in locating specific data points quickly. Consider using the browser's search function (Ctrl+F or Cmd+F) to find specific keys or values in formatted JSON.
Validating JSON
The tool automatically validates your JSON when you paste it or click the "Format JSON" button. If your JSON is valid, you'll see a success message. If not, the tool will highlight the specific error and its location.
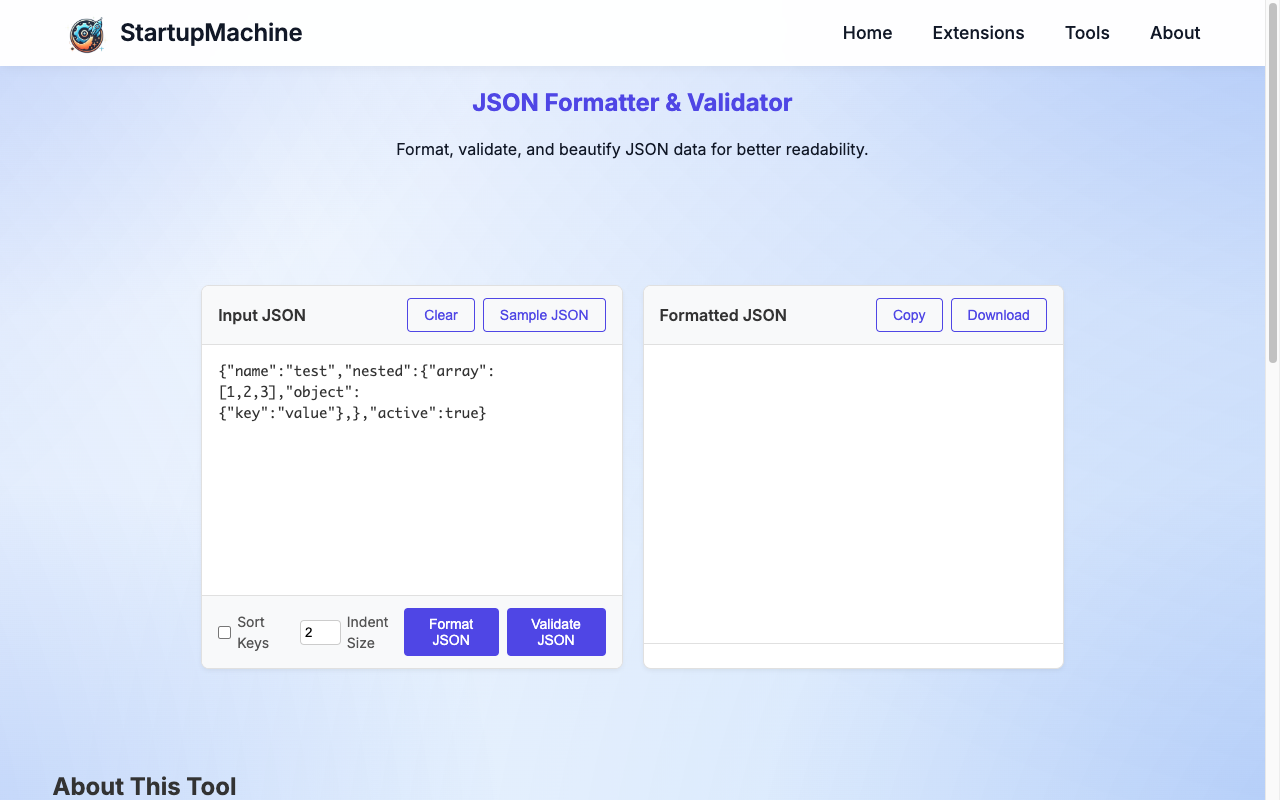
Validation error highlighting a JSON syntax issue
Understanding Error Messages
When JSON validation fails, the tool provides specific error messages that help you identify and fix the problem. Common error messages include:
Error Message | Common Cause | Solution |
---|---|---|
Unexpected token in JSON | Invalid character or syntax error | Check for mismatched quotes or brackets |
Expected property name or '}' | Missing comma or property name | Add the missing comma or properly format the object |
Unexpected end of JSON input | Incomplete JSON structure | Ensure all opened brackets and braces are closed |
Expected ',' or ']' | Missing comma in array or extra comma after last item | Fix array formatting by adding or removing commas |
Expected double-quoted property name | Property names not in double quotes | Ensure all property names are enclosed in double quotes |
Common Mistake
One of the most frequent JSON errors is the presence of trailing commas after the last element in an array or object. While many JavaScript environments allow this, standard JSON does not. Always ensure you don't have an extra comma after the last element.
Copying and Using Formatted JSON
Once your JSON is properly formatted and validated:
- Click the "Copy" button to copy the formatted JSON to your clipboard
- You can now paste it into your code, API testing tool, or documentation
Advanced Features
JSON Minification
For production environments or when working with APIs that have size limitations, you might want to minify your JSON to reduce its size:
- Format your JSON first to ensure it's valid
- Click the "Minify" button to remove all unnecessary whitespace
- The resulting minified JSON will be functionally identical but take up less space
Minified JSON (Same as the example above)
{"users":[{"id":1,"name":"John Smith","email":"[email protected]","active":true,"roles":["admin","editor"]},{"id":2,"name":"Jane Doe","email":"[email protected]","active":false,"roles":["viewer"]}],"total":2,"page":1}
Tree View Visualization
Our tool also provides a tree view option that allows you to visualize the JSON structure hierarchically:
- After formatting your JSON, click on the "Tree View" tab
- The JSON will be displayed as an expandable/collapsible tree
- This is particularly useful for complex nested structures
Advanced Tip
When working with very large JSON files, the tree view can help you navigate through complex structures more efficiently. You can collapse nodes you're not interested in and focus only on the relevant parts of the data.
JSON Best Practices
Structuring Your JSON
- Be Consistent: Use a consistent naming convention for properties (camelCase, snake_case, etc.)
- Keep It Simple: Avoid overly nested structures when possible
- Use Arrays Appropriately: Arrays should contain items of the same type and structure
- Ensure Completeness: Include all required fields in each object
API Response Best Practices
When designing JSON responses for APIs:
- Include metadata about the response (status, version, pagination info)
- Wrap data in descriptive objects (e.g., "users" for a list of users)
- Use consistent error formats
- Consider including hypermedia links for RESTful APIs
Example of Well-Structured API Response
{
"meta": {
"status": "success",
"code": 200,
"page": 1,
"total_pages": 5,
"total_records": 25
},
"data": {
"users": [
{
"id": 101,
"name": "Alice Johnson",
"email": "[email protected]"
},
{
"id": 102,
"name": "Bob Smith",
"email": "[email protected]"
}
]
},
"links": {
"self": "/api/users?page=1",
"next": "/api/users?page=2",
"prev": null
}
}
Troubleshooting Common Errors
Fixing Syntax Errors
Here are solutions to common JSON syntax issues:
Issue | Incorrect | Correct |
---|---|---|
Unquoted property names | {name: "John"} |
{"name": "John"} |
Single quotes | {'name': 'John'} |
{"name": "John"} |
Trailing commas | {"users": ["John", "Jane", ],} |
{"users": ["John", "Jane"]} |
JavaScript comments | {"name": "John" // User name} |
{"name": "John"} |
Missing quotes around strings | {"name": John} |
{"name": "John"} |
Handling Complex JSON
When working with large or complex JSON structures:
- Break it down: Validate smaller portions first if you're having issues with a large file
- Check for encoding issues: Ensure your JSON is properly encoded (UTF-8 is recommended)
- Look for escape sequences: Improperly escaped special characters can cause validation errors
Important
When pasting JSON from external sources, be careful with invisible characters, BOM (Byte Order Mark), or non-standard whitespace characters that can cause validation errors even though the JSON looks correct visually.
Conclusion
Working with JSON is an essential skill for modern web development. Whether you're building APIs, parsing responses, or managing configuration files, having a reliable JSON Formatter & Validator tool can significantly improve your workflow and reduce debugging time.
Our JSON Formatter & Validator tool provides a straightforward way to:
- Format messy or minified JSON for better readability
- Validate JSON structure and syntax
- Identify and fix common JSON errors
- Minify JSON for production environments
- Visualize complex data structures with the tree view
By incorporating this tool into your development workflow, you can ensure your JSON data is always properly structured, valid, and optimized for its intended use.
Ready to Format and Validate Your JSON?
Try our JSON Formatter & Validator now to simplify your development workflow and ensure your JSON is always correct.
Try JSON Formatter & Validator