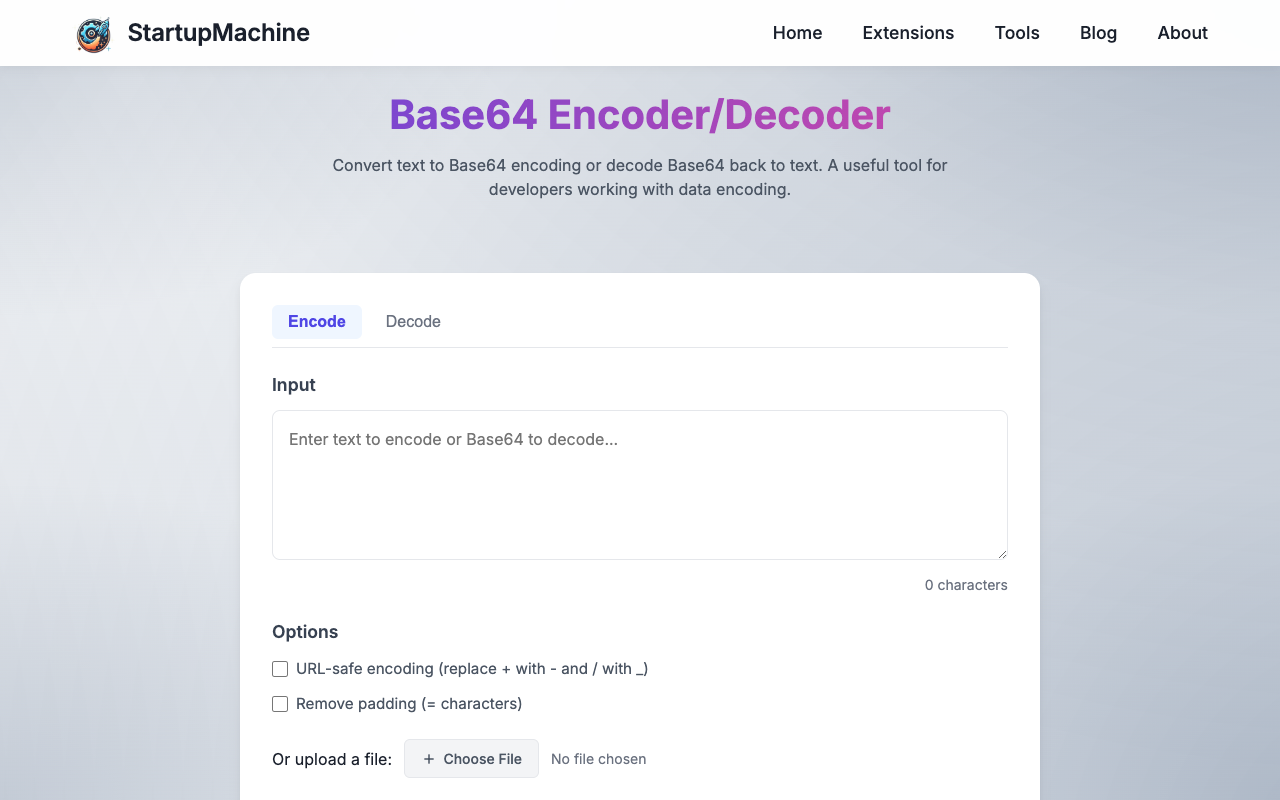
StartupMachine's Base64 Converter tool interface
Base64 encoding is a technique that converts binary data into a text format that can be safely included in HTML, CSS, JavaScript, and other web assets. For web developers, this encoding method provides a convenient way to embed images directly into code, reducing HTTP requests and simplifying deployment in certain scenarios.
In this comprehensive guide, we'll explore how to convert images to Base64 using our free online Base64 Converter tool. We'll also dive into practical use cases, performance considerations, and best practices for implementing Base64 encoding in your web projects.
Key Takeaways
- Learn what Base64 encoding is and when to use it in web development
- Master the step-by-step process of converting images to Base64
- Understand best practices for implementing Base64 encoded images
- Discover performance considerations and optimization techniques
- Explore practical use cases for Base64 encoding in web projects
What is Base64 Encoding?
Base64 is a binary-to-text encoding scheme that represents binary data in an ASCII string format. It uses a set of 64 characters (A-Z, a-z, 0-9, + and /) that are common to most encodings and are printable. This encoding is designed to allow binary data to be transmitted over media that are designed to handle textual data.
When applied to images, Base64 encoding converts the binary image data into a string of text that can be embedded directly into HTML, CSS, or JavaScript files. This eliminates the need for separate image files and reduces the number of HTTP requests required to load a web page.
Technical Note
Base64 encoding increases the size of the original binary data by approximately 33%, as it represents 3 bytes of binary data with 4 ASCII characters. This size increase is an important consideration when deciding whether to use Base64 encoding.
Converting Images to Base64: Step-by-Step Guide
Let's walk through the process of converting an image to Base64 using our Base64 Encoder/Decoder tool:
Step 1: Access the Base64 Converter Tool
Visit our Base64 Converter tool to get started. You'll see an interface that allows for both text and file uploads.
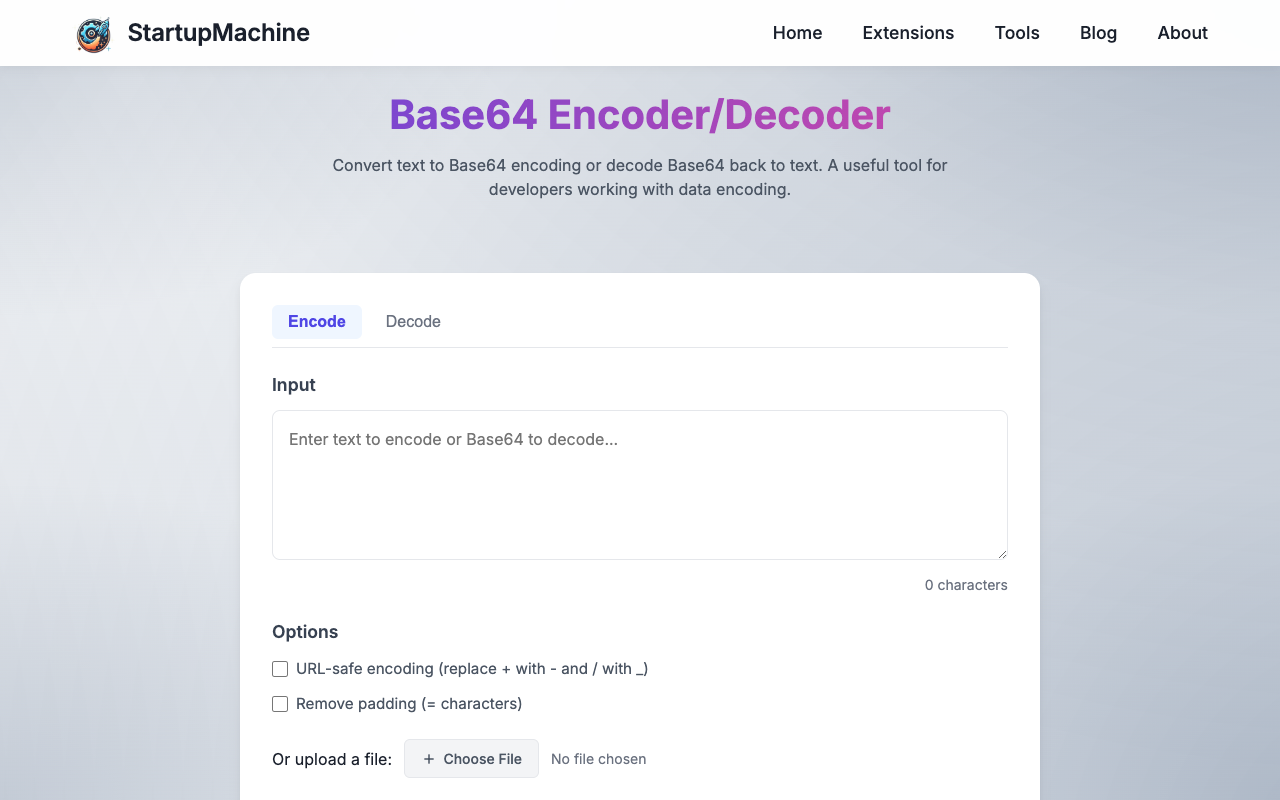
The Base64 Converter tool with file upload and encoding options
Step 2: Upload Your Image
Click on the "Browse" button or drag and drop your image file into the designated area. Our tool supports various image formats including PNG, JPEG, GIF, and SVG.
Step 3: Encode the Image
Once your image is uploaded, click the "Encode" button. The tool will process your image and convert it to a Base64 string.
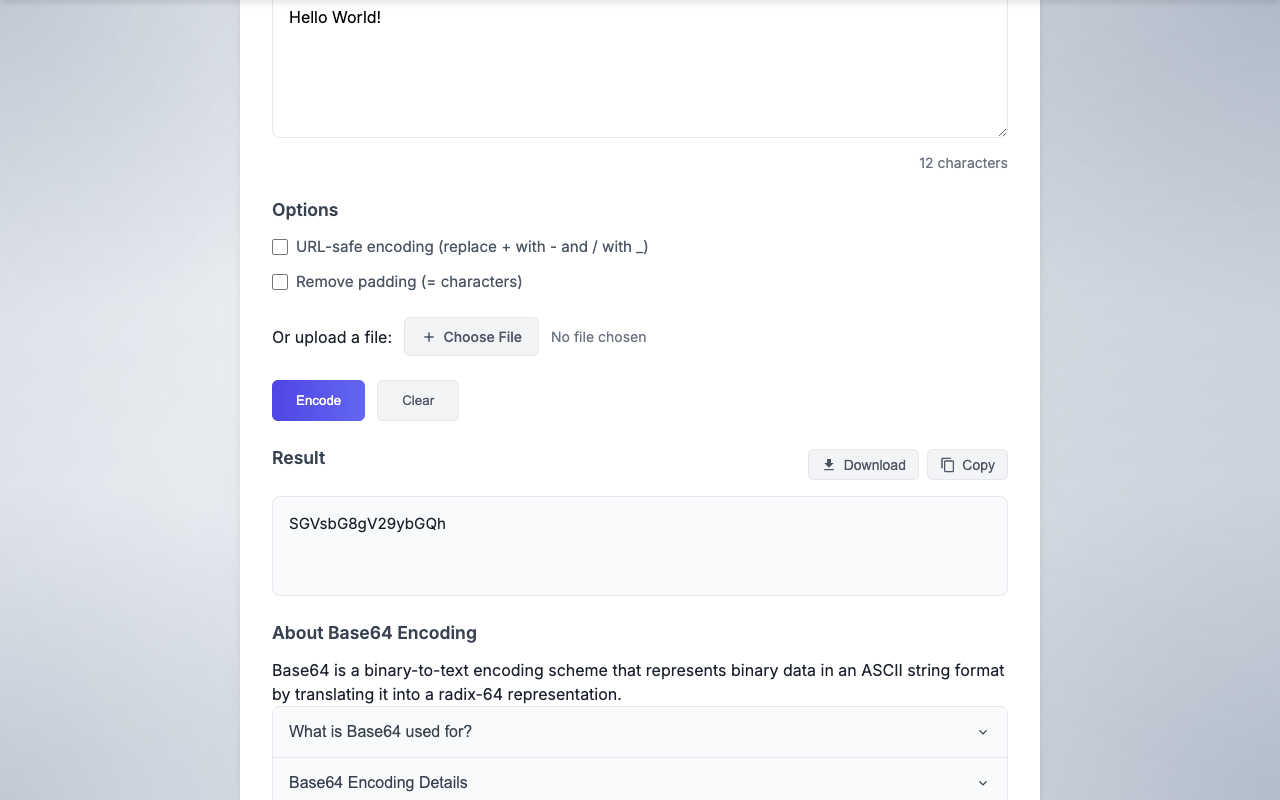
Base64 encoding results after processing an image
Step 4: Copy the Base64 String
After the encoding process is complete, you'll see the Base64 string in the output area. Click the "Copy" button to copy the encoded string to your clipboard. You can also download the result as a text file if needed.
Step 5: Implement in Your Web Project
Now you can use this Base64 string in your HTML, CSS, or JavaScript code. Here's an example of how to use it in different contexts:
HTML Implementation
<img src="data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAA...YOUR_BASE64_STRING..." alt="Base64 encoded image">
CSS Implementation
.element-with-bg-image {
background-image: url('data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAA...YOUR_BASE64_STRING...');
}
JavaScript Implementation
const img = new Image();
img.src = 'data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAA...YOUR_BASE64_STRING...';
document.body.appendChild(img);
Decoding Base64 Back to Images
Our tool also supports decoding Base64 strings back to images. This can be useful for testing or extracting images from existing code:
Step 1: Paste Your Base64 String
Enter the Base64 encoded string into the input area of the tool. Make sure to include only the Base64 part, not the data URI prefix (data:image/png;base64,).
Step 2: Click "Decode"
Click the "Decode" button to convert the Base64 string back to an image.
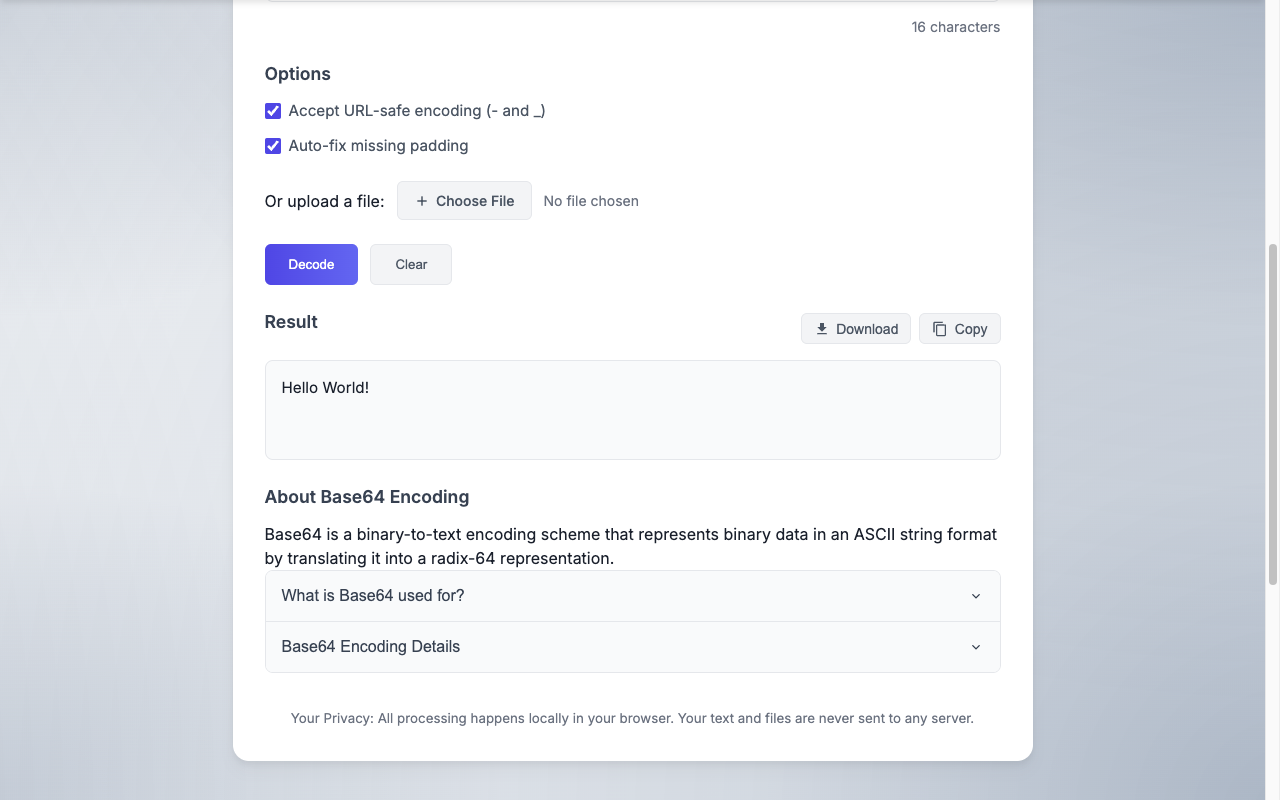
The Base64 Converter tool displaying a decoded image from a Base64 string
Step 3: Download or View the Image
After decoding, you can view the image directly in the tool and download it to your computer if needed.
Practical Use Cases for Base64 Images
Base64 encoding images can be beneficial in several scenarios:
1. Small Icons and UI Elements
Small icons, logos, or UI elements that are frequently used throughout your website can benefit from Base64 encoding. This reduces HTTP requests and can improve page load times.
2. Single-File Applications
For HTML emails, offline documentation, or single-file web applications, embedding images as Base64 strings ensures that all visual elements are available without external dependencies.
3. Reducing HTTP Requests
Each image on a webpage typically requires a separate HTTP request. By embedding small images directly into your HTML or CSS using Base64, you can reduce the number of requests and potentially improve load times.
4. SVG Embedding
SVG files, which are already text-based, can be Base64 encoded and embedded into CSS or HTML, allowing for easy scaling and manipulation while reducing HTTP requests.
5. Data URIs in Canvas Applications
When working with the HTML5 Canvas element, Base64 data URIs can be used to load images directly into the canvas without requiring separate image files.
Performance Considerations
While Base64 encoding offers advantages in certain scenarios, it's important to consider the following performance implications:
Performance Alert
Base64 encoding increases file size by approximately 33%, which can offset the benefits of reducing HTTP requests. Use Base64 encoding judiciously.
File Size Increase
As mentioned earlier, Base64 encoding increases the size of the original binary data by about 33%. This means that your HTML, CSS, or JavaScript files will be larger when they contain Base64 encoded images.
Caching Implications
Browser caching works differently for embedded Base64 images compared to separate image files:
- Individual image files can be cached independently and reused across multiple pages.
- Base64 embedded images are cached as part of the document they're embedded in.
Parsing Overhead
Browsers need to parse and decode Base64 strings before they can render the images, which adds a small processing overhead.
When to Use Base64
Based on these considerations, here are general guidelines for when to use Base64 encoding:
Use Base64 | Avoid Base64 |
---|---|
Small images (under 10KB) | Large images |
Infrequently updated images | Frequently changed images |
Icons and simple UI elements | Photos and complex graphics |
Self-contained applications | Websites with robust caching |
Best Practices for Using Base64 Images
To maximize the benefits of Base64 encoding while minimizing drawbacks, follow these best practices:
1. Optimize Images Before Encoding
Always optimize your images before converting them to Base64. Use tools like our Image Compressor to reduce file size without significant quality loss.
2. Use for Small, Critical Assets Only
Limit Base64 encoding to small, critical assets that need to appear immediately during page load, such as logos and essential UI elements.
3. Consider HTTP/2 Implications
With HTTP/2's multiplexing capabilities, the benefits of reducing HTTP requests through Base64 encoding are less significant. If your site uses HTTP/2, you might get better performance by keeping images as separate files.
4. Measure Performance Impact
Always measure the performance impact of Base64 encoding using tools like Google Lighthouse or WebPageTest to ensure it's providing the expected benefits.
5. Implement Conditional Loading
Consider implementing conditional loading strategies where Base64 is used for initial page load, but larger, separate image files are loaded for subsequent visits or when bandwidth allows.
Conclusion
Base64 encoding provides web developers with a valuable technique for embedding binary data directly into web documents. When used appropriately—for small, critical assets—it can improve performance by reducing HTTP requests and simplifying deployment.
Our free Base64 Converter tool makes it easy to encode and decode images, allowing you to seamlessly integrate Base64 encoded assets into your web projects.
Remember to consider the performance implications of Base64 encoding and follow the best practices outlined in this guide to ensure you're using this technique effectively in your web development workflow.
Ready to Convert Your Images to Base64?
Visit our Base64 Converter to easily encode and decode images for your web development projects.
Try Base64 Converter